11-13 5 views
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 |
#!/usr/bin/env python # -*- coding: utf-8 -*- import os import json import tempfile import requests_cache class Market(object): _session = None __DEFAULT_BASE_URL = 'https://api.coinmarketcap.com/v2/' __DEFAULT_TIMEOUT = 30 __TEMPDIR_CACHE = True def __init__(self, base_url=__DEFAULT_BASE_URL, request_timeout=__DEFAULT_TIMEOUT, tempdir_cache=__TEMPDIR_CACHE): self.base_url = base_url self.request_timeout = request_timeout self.cache_filename = 'coinmarketcap_cache' self.cache_name = os.path.join(tempfile.gettempdir(), self.cache_filename) if tempdir_cache else self.cache_filename @property def session(self): if not self._session: self._session = requests_cache.core.CachedSession(cache_name=self.cache_name, backend='sqlite', expire_after=120) self._session.headers.update({'Content-Type': 'application/json'}) self._session.headers.update({'User-agent': 'coinmarketcap - python wrapper around coinmarketcap.com (github.com/barnumbirr/coinmarketcap)'}) return self._session def __request(self, endpoint, params): response_object = self.session.get(self.base_url + endpoint, params=params, timeout=self.request_timeout) try: response = json.loads(response_object.text) if isinstance(response, list) and response_object.status_code == 200: response = [dict(item, **{u'cached':response_object.from_cache}) for item in response] if isinstance(response, dict) and response_object.status_code == 200: response[u'cached'] = response_object.from_cache except Exception as e: return e return response def listings(self): """ This endpoint displays all active cryptocurrency listings in one call. Use the "id" field on the Ticker endpoint to query more information on a specific cryptocurrency. """ response = self.__request('listings/', params=None) return response def ticker(self, currency="", **kwargs): """ This endpoint displays cryptocurrency ticker data in order of rank. The maximum number of results per call is 100. Pagination is possible by using the start and limit parameters. GET /ticker/ Optional parameters: (int) start - return results from rank [start] and above (default is 1) (int) limit - return a maximum of [limit] results (default is 100; max is 100) (string) convert - return pricing info in terms of another currency. Valid fiat currency values are: "AUD", "BRL", "CAD", "CHF", "CLP", "CNY", "CZK", "DKK", "EUR", "GBP", "HKD", "HUF", "IDR", "ILS", "INR", "JPY", "KRW", "MXN", "MYR", "NOK", "NZD", "PHP", "PKR", "PLN", "RUB", "SEK", "SGD", "THB", "TRY", "TWD", "ZAR" Valid cryptocurrency values are: "BTC", "ETH" "XRP", "LTC", and "BCH" GET /ticker/{id} Optional parameters: (string) convert - return pricing info in terms of another currency. Valid fiat currency values are: "AUD", "BRL", "CAD", "CHF", "CLP", "CNY", "CZK", "DKK", "EUR", "GBP", "HKD", "HUF", "IDR", "ILS", "INR", "JPY", "KRW", "MXN", "MYR", "NOK", "NZD", "PHP", "PKR", "PLN", "RUB", "SEK", "SGD", "THB", "TRY", "TWD", "ZAR" Valid cryptocurrency values are: "BTC", "ETH" "XRP", "LTC", and "BCH" """ params = {} params.update(kwargs) # see https://github.com/barnumbirr/coinmarketcap/pull/28 if currency: currency = str(currency) + '/' response = self.__request('ticker/' + currency, params) return response def stats(self, **kwargs): """ This endpoint displays the global data found at the top of coinmarketcap.com. Optional parameters: (string) convert - return pricing info in terms of another currency. Valid fiat currency values are: "AUD", "BRL", "CAD", "CHF", "CLP", "CNY", "CZK", "DKK", "EUR", "GBP", "HKD", "HUF", "IDR", "ILS", "INR", "JPY", "KRW", "MXN", "MYR", "NOK", "NZD", "PHP", "PKR", "PLN", "RUB", "SEK", "SGD", "THB", "TRY", "TWD", "ZAR" Valid cryptocurrency values are: "BTC", "ETH" "XRP", "LTC", and "BCH" """ params = {} params.update(kwargs) response = self.__request('global/', params) return response |
使用
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 |
coinmarketcap = Market() tickersearch_dict = coinmarketcap.ticker(tickersearch_instance.tickerid) if tickersearch_dict['metadata']['error']: logger.warning("获取代币{0}数据错误,错误内容:{1}".format(tickersearch_instance.symbol, tickersearch_instance['metadata']['error'])) else: tickersearch_instance.rank = tickersearch_dict['data']['rank'] tickersearch_instance.circulating_supply = tickersearch_dict['data']['circulating_supply'] tickersearch_instance.total_supply = tickersearch_dict['data']['total_supply'] tickersearch_instance.max_supply = tickersearch_dict['data']['max_supply'] tickersearch_instance.symbol = tickersearch_dict['data']['symbol'] tickersearch_instance.name = tickersearch_dict['data']['name'] tickersearch_instance.website_slug = tickersearch_dict['data']['website_slug'] tickersearch_instance.save(casecade=False) logger.info("成功获取 TICKER:{0}: 名称:{1}, 简称:{2}, 笔名:{3}, 市值排名:{4}, 总供应:{5}, 循环供应:{6}, 最大供应:{7}".format( tickersearch_instance.symbol, tickersearch_instance.name, tickersearch_instance.symbol, tickersearch_instance.website_slug, tickersearch_instance.rank, tickersearch_instance.total_supply, tickersearch_instance.circulating_supply, tickersearch_instance.max_supply)) |
如果想赏钱,可以用微信扫描下面的二维码,一来能刺激我写博客的欲望,二来好维护云主机的费用; 另外再次标注博客原地址 itnotebooks.com 感谢!
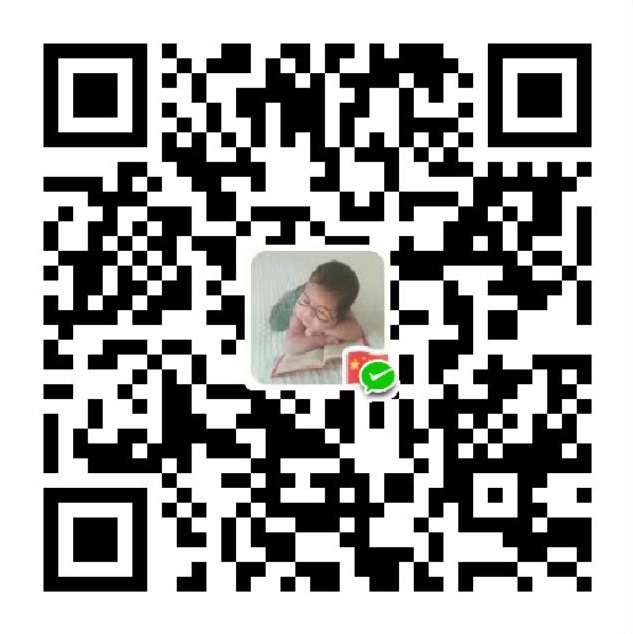