11-08 13 views
本文中是以h3c的为例,其它品牌的请自行扩展
另本文中是以查询信息相关信息为例,换成查看配置的命令即可起到备份配置的作用
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 |
#!/usr/bin/env python3 # -*- coding: utf-8 -*- # @Author : Eric Winn # @Email : eng.eric.winn@gmail.com # @Time : 2018/11/8 9:15 # @Version : 1.0 # @File : switch_telnet.py # @Software : PyCharm import telnetlib import time from multiprocessing import Process import datetime now = datetime.datetime.now() # 将结果定入到文件中 def Output(ip, msg): # 输出时的文件名 filename = "{0}_{1}-{2}-{3}_{4}-{5}-{6}.log".format(ip, now.year, now.month, now.day, now.hour, now.minute, now.second) print('====>{}'.format(filename)) time.sleep(.1) try: fp = open(filename, "w") fp.write(msg) except Exception, e: print(e) return 1 fp.close() return 0 # Factory继承Process,多进程 class TelnetClient(Process): def __init__(self, host, username, password): Process.__init__(self) self.host = host self.username = username self.password = password def run(self): try: # 建立连接 tn = telnetlib.Telnet(self.host, port=23, timeout=5) tn.set_debuglevel(5) tn.read_until(b"Username:", timeout=2) tn.write(self.username + b"\n") tn.read_until(b"Password:", timeout=2) tn.write(self.password + b"\n") tn.write(b"\n") time.sleep(1) output = "" for cmds in open(r'cmds.txt', 'r').readlines(): cmd = cmds.strip('\n') tn.write(cmd + b'\n') # 不接收除dis外的其它命令 if cmd in ['dis cpu', 'dis memory', 'dis pow', 'dis fan', 'dis envi', 'dis version']: i = True time.sleep(10) output += "\r\n" # 解决多屏输出问题 while i: # 如果输出是以'---- More ----'结尾,则输入空格换屏 ret = tn.read_until('---- More ----', 5) if ret.endswith('---- More ----'): # 将本次的输出结果去'---- More ----'读入到output中 output += ret.strip('---- More ----') for i in range(10): # 输入空格 tn.write(' ') time.sleep(0.1) else: # 不是以'---- More ----'结尾,保存本次输出到output中并结束while循环 output += ret tn.write("\r\n") i = False else: time.sleep(10) tn.write("\r\n") time.sleep(10) output += tn.read_very_eager() # 输将结果出到文件 ret = Output(self.host, output) if ret == 1: return 1 except: print("Can't connection %s" % self.host) return if __name__ == "__main__": username = "monitor" password = "123456" for host in open('switch_list.txt').readlines(): client = TelnetClient(host.strip('\n'), username, password) client.start() |
如果想赏钱,可以用微信扫描下面的二维码,一来能刺激我写博客的欲望,二来好维护云主机的费用; 另外再次标注博客原地址 itnotebooks.com 感谢!
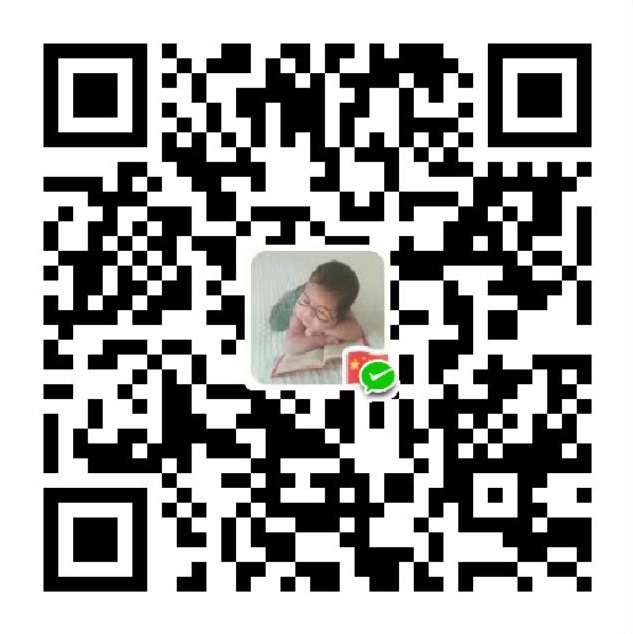
我用的pycharm断点测试,到了
def run(self):
try:
就不往下执行了,直接跳到最后了,请问什么情况呢?
有报错,请问大佬如何处理,谢谢!我用的python 3.8
Telnet(10.1.8.5,23): recv b’************************************************\r\n’
Telnet(10.1.8.5,23): recv b’* Copyright (c) 2004-2016 Hangzhou H3C Tech. Co., ‘
Telnet(10.1.8.5,23): recv b”Ltd. All rights reserved. *\r\n* Without the owner’”
Telnet(10.1.8.5,23): recv b’s prior written consent, ‘
Telnet(10.1.8.5,23): recv b’ *\r\n* no decompiling or reverse-engineering ‘
Telnet(10.1.8.5,23): recv b’shall be allowed. *\r\n**********’
Telnet(10.1.8.5,23): recv b’**************************************************’
Telnet(10.1.8.5,23): recv b’******************\r\n’
Telnet(10.1.8.5,23): recv b’\xff\xfc\x01′
Telnet(10.1.8.5,23): IAC WONT 1
Telnet(10.1.8.5,23): recv b’\xff\xfc\x03′
Telnet(10.1.8.5,23): IAC WONT 3
Telnet(10.1.8.5,23): recv b’\xff\xfe\x18′
Telnet(10.1.8.5,23): IAC DONT 24
Telnet(10.1.8.5,23): recv b’\xff\xfe\x1f’
Telnet(10.1.8.5,23): IAC DONT 31
Telnet(10.1.8.5,23): recv b’\r\n\r\nLogin authentication\r\n\r\n’
Telnet(10.1.8.5,23): recv b’\r\nUsername:’
Can’t connection 10.1.8.5
有报错,请问大佬如何处理,谢谢!我用的python 3.8
Telnet(10.1.8.5,23): recv b’************************************************\r\n’
Telnet(10.1.8.5,23): recv b’* Copyright (c) 2004-2016 Hangzhou H3C Tech. Co., ‘
Telnet(10.1.8.5,23): recv b”Ltd. All rights reserved. *\r\n* Without the owner'”
Telnet(10.1.8.5,23): recv b’s prior written consent, ‘
Telnet(10.1.8.5,23): recv b’ *\r\n* no decompiling or reverse-engineering ‘
Telnet(10.1.8.5,23): recv b’shall be allowed. *\r\n**********’
Telnet(10.1.8.5,23): recv b’**************************************************’
Telnet(10.1.8.5,23): recv b’******************\r\n’
Telnet(10.1.8.5,23): recv b’\xff\xfc\x01′
Telnet(10.1.8.5,23): IAC WONT 1
Telnet(10.1.8.5,23): recv b’\xff\xfc\x03′
Telnet(10.1.8.5,23): IAC WONT 3
Telnet(10.1.8.5,23): recv b’\xff\xfe\x18′
Telnet(10.1.8.5,23): IAC DONT 24
Telnet(10.1.8.5,23): recv b’\xff\xfe\x1f’
Telnet(10.1.8.5,23): IAC DONT 31
Telnet(10.1.8.5,23): recv b’\r\n\r\nLogin authentication\r\n\r\n’
Telnet(10.1.8.5,23): recv b’\r\nUsername:’
Can’t connection 10.1.1.1
“D:\Program Files (x86)\python3.6\python.exe” D:/desktop/python3.6/1.py
Telnet(192.168.10.254,23): recv b’\r\r\nWarning: Telnet is not a secure protocol, and i’
Telnet(192.168.10.254,23): recv b’t is recommended to use Stelnet.\r\n\r\nLogin authenti’
Telnet(192.168.10.254,23): recv b’cation\r\n\r\n\r\nUsername:\xff\xfb\x01\xff\xfb\x01\xff\xfb\x01\xff\xfb\x03\xff\xfd\x18\xff\xfd\x1f’
Telnet(192.168.10.254,23): IAC WILL 1
Telnet(192.168.10.254,23): IAC WILL 1
Telnet(192.168.10.254,23): IAC WILL 1
Telnet(192.168.10.254,23): IAC WILL 3
Telnet(192.168.10.254,23): IAC DO 24
Telnet(192.168.10.254,23): IAC DO 31
Can’t connection 192.168.10.254
有报错哦,python版本是3.6。希望大佬能够不吝赐教 😀
出现了类型报错。
贴出来看看