7-30 14 views
背景
公司使用的是网宿的cdn平台,考虑开发经常要运维部门这边帮忙刷新。就寻思的着做成一个页面,可以让他们自行去操作。
网宿官网是只提供了shell版的demo,就利用了茶余饭后的时间写了一个python版的好做成一个页面,废话不多说,上代码
代码
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 |
#!/usr/bin/env python # -*- coding: utf-8 -*- # @Author : Eric Winn # @Email : eng.eric.winn@gmail.com # @Time : 2018-05-18 12:15 # @Version : 1.0 # @File : cdn_flush # @Software : PyCharm import requests import json import datetime import hmac import hashlib import base64 import sys # auth username = "XXX" apiKey = b"XXXXXXXXX" # domain domain="http://www.abc.com/" # time now = datetime.datetime.now() nowTime = now.strftime('%a, %d %b %Y %H:%M:%S GMT') nowTime_bytes = bytes(nowTime, encoding='utf-8') # headers headers = { "Content-type": "application/json", "Date": nowTime } # token value = hmac.new(apiKey, nowTime_bytes, hashlib.sha1).digest() token = base64.b64encode(value).rstrip() # api url url = "https://open.chinanetcenter.com/ccm/purge/ItemIdReceiver" # post data data = json.dumps({ "dirs": [ domain ], }) # post r = requests.post(url, data=data, headers=headers, auth=(username, token)) result = json.loads(r.text) print(result) |
如果想赏钱,可以用微信扫描下面的二维码,一来能刺激我写博客的欲望,二来好维护云主机的费用; 另外再次标注博客原地址 itnotebooks.com 感谢!
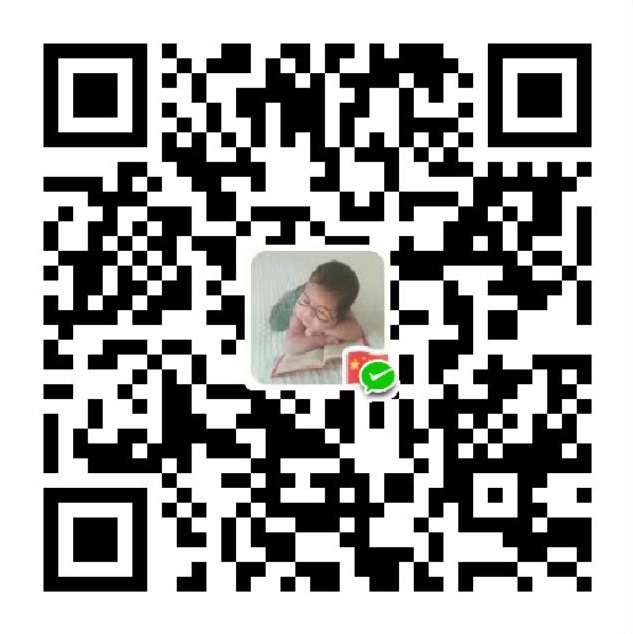