6-10 1 views
利用Terraform实现运维平台的IaaS层,由于公司用的全是阿里云,所以以阿里云为例,不过既然是Terraform,其实无需太在意这个,基类封装好,什么云可以,甚至是VSphere
本文中只例出写个比较重要的代码块
系统中需要提前安装好Terraform,虽然是Python库其实是“subprocess.Popen”执行的系统命令
以下为Terraform Python库的源码
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 131 132 133 134 135 136 137 138 139 140 141 142 143 144 145 146 147 148 149 150 151 152 153 154 155 156 157 158 159 160 161 162 163 164 165 166 167 168 169 170 171 172 173 174 175 176 177 178 179 180 181 182 183 184 185 186 187 188 189 190 191 192 193 194 195 196 197 198 199 200 201 202 203 204 205 206 207 208 209 210 211 212 213 214 215 216 217 218 219 220 221 222 223 224 225 226 227 228 229 230 231 232 233 234 235 236 237 238 239 240 241 242 243 244 245 246 247 248 249 250 251 252 253 254 255 256 257 258 259 260 261 262 263 264 265 266 267 268 269 270 271 272 273 274 275 276 277 278 279 280 281 282 283 284 285 286 287 288 |
class Terraform(object): """ Wrapper of terraform command line tool https://www.terraform.io/ """ def __init__(self, working_dir=None, targets=None, state=None, variables=None, parallelism=None, var_file=None, terraform_bin_path=None, is_env_vars_included=True, ): """ :param working_dir: the folder of the working folder, if not given, will be current working folder :param targets: list of target as default value of apply/destroy/plan command :param state: path of state file relative to working folder, as a default value of apply/destroy/plan command :param variables: default variables for apply/destroy/plan command, will be override by variable passing by apply/destroy/plan method :param parallelism: default parallelism value for apply/destroy command :param var_file: passed as value of -var-file option, could be string or list, list stands for multiple -var-file option :param terraform_bin_path: binary path of terraform :type is_env_vars_included: bool :param is_env_vars_included: included env variables when calling terraform cmd """ self.is_env_vars_included = is_env_vars_included self.working_dir = working_dir self.state = state self.targets = [] if targets is None else targets self.variables = dict() if variables is None else variables self.parallelism = parallelism self.terraform_bin_path = terraform_bin_path \ if terraform_bin_path else 'terraform' self.var_file = var_file self.temp_var_files = VariableFiles() # store the tfstate data self.tfstate = None self.read_state_file(self.state) def __getattr__(self, item): def wrapper(*args, **kwargs): cmd_name = str(item) if cmd_name.endswith('_cmd'): cmd_name = cmd_name[:-4] logging.debug('called with %r and %r' % (args, kwargs)) return self.cmd(cmd_name, *args, **kwargs) return wrapper def apply(self, dir_or_plan=None, input=False, skip_plan=False, no_color=IsFlagged, **kwargs): """ refer to https://terraform.io/docs/commands/apply.html no-color is flagged by default :param no_color: disable color of stdout :param input: disable prompt for a missing variable :param dir_or_plan: folder relative to working folder :param skip_plan: force apply without plan (default: false) :param kwargs: same as kwags in method 'cmd' :returns return_code, stdout, stderr """ default = kwargs default['input'] = input default['no_color'] = no_color default['auto-approve'] = (skip_plan == True) option_dict = self._generate_default_options(default) args = self._generate_default_args(dir_or_plan) # 将组好的命令参数做为入参调用封装好的cmd函数 return self.cmd('apply', *args, **option_dict) def _generate_default_args(self, dir_or_plan): return [dir_or_plan] if dir_or_plan else [] def _generate_default_options(self, input_options): option_dict = dict() option_dict['state'] = self.state option_dict['target'] = self.targets option_dict['var'] = self.variables option_dict['var_file'] = self.var_file option_dict['parallelism'] = self.parallelism option_dict['no_color'] = IsFlagged option_dict['input'] = False option_dict.update(input_options) return option_dict def destroy(self, dir_or_plan=None, force=IsFlagged, **kwargs): """ refer to https://www.terraform.io/docs/commands/destroy.html force/no-color option is flagged by default :return: ret_code, stdout, stderr """ default = kwargs default['force'] = force options = self._generate_default_options(default) args = self._generate_default_args(dir_or_plan) return self.cmd('destroy', *args, **options) def plan(self, dir_or_plan=None, detailed_exitcode=IsFlagged, **kwargs): """ refer to https://www.terraform.io/docs/commands/plan.html :param detailed_exitcode: Return a detailed exit code when the command exits. :param dir_or_plan: relative path to plan/folder :param kwargs: options :return: ret_code, stdout, stderr """ options = kwargs options['detailed_exitcode'] = detailed_exitcode options = self._generate_default_options(options) args = self._generate_default_args(dir_or_plan) return self.cmd('plan', *args, **options) def init(self, dir_or_plan=None, backend_config=None, reconfigure=IsFlagged, backend=True, **kwargs): """ refer to https://www.terraform.io/docs/commands/init.html By default, this assumes you want to use backend config, and tries to init fresh. The flags -reconfigure and -backend=true are default. :param dir_or_plan: relative path to the folder want to init :param backend_config: a dictionary of backend config options. eg. t = Terraform() t.init(backend_config={'access_key': 'myaccesskey', 'secret_key': 'mysecretkey', 'bucket': 'mybucketname'}) :param reconfigure: whether or not to force reconfiguration of backend :param backend: whether or not to use backend settings for init :param kwargs: options :return: ret_code, stdout, stderr """ options = kwargs options['backend_config'] = backend_config options['reconfigure'] = reconfigure options['backend'] = backend options = self._generate_default_options(options) args = self._generate_default_args(dir_or_plan) return self.cmd('init', *args, **options) def generate_cmd_string(self, cmd, *args, **kwargs): """ for any generate_cmd_string doesn't written as public method of terraform examples: 1. call import command, ref to https://www.terraform.io/docs/commands/import.html --> generate_cmd_string call: terraform import -input=true aws_instance.foo i-abcd1234 --> python call: tf.generate_cmd_string('import', 'aws_instance.foo', 'i-abcd1234', input=True) 2. call apply command, --> generate_cmd_string call: terraform apply -var='a=b' -var='c=d' -no-color the_folder --> python call: tf.generate_cmd_string('apply', the_folder, no_color=IsFlagged, var={'a':'b', 'c':'d'}) :param cmd: command and sub-command of terraform, seperated with space refer to https://www.terraform.io/docs/commands/index.html :param args: arguments of a command :param kwargs: same as kwags in method 'cmd' :return: string of valid terraform command """ cmds = cmd.split() # 拼接上terraform命令的绝对路径或terraform命令 cmds = [self.terraform_bin_path] + cmds for option, value in kwargs.items(): if '_' in option: option = option.replace('_', '-') if type(value) is list: for sub_v in value: cmds += ['-{k}={v}'.format(k=option, v=sub_v)] continue if type(value) is dict: if 'backend-config' in option: for bk, bv in value.items(): cmds += ['-backend-config={k}={v}'.format(k=bk, v=bv)] continue # since map type sent in string won't work, create temp var file for # variables, and clean it up later else: filename = self.temp_var_files.create(value) cmds += ['-var-file={0}'.format(filename)] continue # simple flag, if value is IsFlagged: cmds += ['-{k}'.format(k=option)] continue if value is None or value is IsNotFlagged: continue if type(value) is bool: value = 'true' if value else 'false' cmds += ['-{k}={v}'.format(k=option, v=value)] cmds += args return cmds def cmd(self, cmd, *args, **kwargs): """ run a terraform command, if success, will try to read state file :param cmd: command and sub-command of terraform, seperated with space refer to https://www.terraform.io/docs/commands/index.html :param args: arguments of a command :param kwargs: any option flag with key value without prefixed dash character if there's a dash in the option name, use under line instead of dash, ex. -no-color --> no_color if it's a simple flag with no value, value should be IsFlagged ex. cmd('taint', allow_missing=IsFlagged) if it's a boolean value flag, assign True or false if it's a flag could be used multiple times, assign list to it's value if it's a "var" variable flag, assign dictionary to it if a value is None, will skip this option if the option 'capture_output' is passed (with any value other than True), terraform output will be printed to stdout/stderr and "None" will be returned as out and err. if the option 'raise_on_error' is passed (with any value that evaluates to True), and the terraform command returns a nonzerop return code, then a TerraformCommandError exception will be raised. The exception object will have the following properties: returncode: The command's return code out: The captured stdout, or None if not captured err: The captured stderr, or None if not captured :return: ret_code, out, err """ capture_output = kwargs.pop('capture_output', True) raise_on_error = kwargs.pop('raise_on_error', False) if capture_output is True: stderr = subprocess.PIPE stdout = subprocess.PIPE else: stderr = sys.stderr stdout = sys.stdout # 拼接Terraform命令 cmds = self.generate_cmd_string(cmd, *args, **kwargs) log.debug('command: {c}'.format(c=' '.join(cmds))) working_folder = self.working_dir if self.working_dir else None environ_vars = {} if self.is_env_vars_included: environ_vars = os.environ.copy() # 执行terrafrom命令 p = subprocess.Popen(cmds, stdout=stdout, stderr=stderr, cwd=working_folder, env=environ_vars) synchronous = kwargs.pop('synchronous', True) if not synchronous: return p, None, None out, err = p.communicate() ret_code = p.returncode log.debug('output: {o}'.format(o=out)) if ret_code == 0: self.read_state_file() else: log.warn('error: {e}'.format(e=err)) self.temp_var_files.clean_up() if capture_output is True: out = out.decode('utf-8') err = err.decode('utf-8') else: out = None err = None if ret_code != 0 and raise_on_error: raise TerraformCommandError( ret_code, ' '.join(cmds), out=out, err=err) return ret_code, out, err |
generate_terraform_file
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 |
from jinja2 import FileSystemLoader, Environment def generate_file_from_string(str, dest, target): """ 将string渲染成文件 :param str: :param dest: :param target: :return: """ if not os.path.exists(target): os.makedirs(target) file = os.path.join(target, dest) try: with open(file, 'w') as f: f.write(str) return os.path.dirname(file) except Exception as e: logger.error(e, exc_info=True) return def generate_terraform_file(target_path, cloud_path, cluster_name, mixin_vars): terraform_path = os.path.join(cloud_path, cluster_name, "terraform") # 渲染模板文件 # Variables.tf: 变量模板 # terraform.tf.j2: ECS实例创建模板 # 指定会用到的几个模板文件需要进行渲染 for tf_file in ['Variables.tf', 'terraform.tf.j2']: _, tf_path = terraform_path.split('{}/'.format(PROJECT_DIR)) variables_content_objs = TerraformCloudProviderTemplate.objects.filter(filename=tf_file, path=tf_path) if not variables_content_objs: return ret = generate_file_from_string(variables_content_objs[0].template, tf_file, terraform_path) if not ret: return # 简单的系统命令的封装 ret_code = send_cmd( ['cp', '-rf', os.path.join(terraform_path, 'Variables.tf'), os.path.join(target_path, 'Variables.tf')]) if ret_code[0] != 0: logger.error(ret_code) return try: lorder = FileSystemLoader(terraform_path) env = Environment(loader=lorder) _template = env.get_template("terraform.tf.j2") result = _template.render(mixin_vars) except Exception as e: logger.error(e, exc_info=True) return ret = generate_file_from_string(result, 'main.tf', target_path) if not ret: return def create_terrafrom_working_dir(cluster_name, task_id): if not os.path.exists(TERRAFORM_DIR): os.makedirs(TERRAFORM_DIR) cluster_dir = os.path.join(TERRAFORM_DIR, cluster_name, task_id) if not os.path.exists(cluster_dir): os.makedirs(cluster_dir) return cluster_dir |
封装的Terraform基类
这里用到了ABCMeta类,强制指定必须复写类似于terraform init之类的关键步骤
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 |
#!/usr/bin/env python3 # -*- coding: utf-8 -*- # @Author : Eric Winn # @Email : eng.eric.winn@gmail.com # @Time : 2020/2/20 4:12 PM # @Version : 1.0 # @File : cloud_client # @Software : PyCharm from abc import ABCMeta, abstractmethod from python_terraform import Terraform, IsNotFlagged from .utils import generate_terraform_file CLOUDS_RESOURCE_DIR = '' class CloudClient(metaclass=ABCMeta): cloud_config_path = CLOUDS_RESOURCE_DIR working_path = None t = None def __init__(self, vars): self.vars = vars @abstractmethod def list_region(self): pass @abstractmethod def init_terraform(self, cluster_name, task): pass @abstractmethod def create_image(self, zone): pass def destroy_terraform(self, cluster): pass def render_template(self, cluster_name, mixed_vars, task): generate_terraform_file(self.working_path, self.cloud_config_path, cluster_name, mixed_vars) def create_terraform(self): self.t = Terraform(working_dir=self.working_path) def plan_terraform(self): ret_code, _, _ = self.t.plan(var=self.vars) print('out:{}'.format(_)) return ret_code def apply_terraform(self): ret_code, out, err = self.t.apply('./', var=self.vars, refresh=True, skip_plan=True, no_color=IsNotFlagged) if ret_code != 0: print('out:{}'.format(out)) print('err:{}'.format(err)) else: print('out:{}'.format(out)) print('err:{}'.format(err)) return ret_code, out, err |
继承CloudClient基类
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 |
#!/usr/bin/env python3 # -*- coding: utf-8 -*- # @Author : Eric Winn # @Email : eng.eric.winn@gmail.com # @Time : 2020/2/21 2:39 AM # @Version : 1.0 # @File : aliyun # @Software : PyCharm import logging import os from.cloud_client import CloudClient from common.models import Setting from django.conf import settings from .utils import download_plugins, create_terrafrom_working_dir logger = logging.getLogger(__file__) class AliCloudClient(CloudClient): cloud_config_path = os.path.join(settings.CLOUDS_RESOURCE_DIR, 'alicloud') working_path = None def list_region(self): pass def list_zone(self, region): pass def create_image(self, zone): pass def init_terraform(self, cluster_name, task): if not self.working_path: self.working_path = create_terrafrom_working_dir(cluster_name=cluster_name, task_id=task['id']) plugin_dir = os.path.join(self.working_path, '.terraform', 'plugins') if not os.path.exists(plugin_dir): os.makedirs(plugin_dir) hostname = settings.MAIN_HOST_NAME url = f"{hostname}/download/provider/alicloud/alicloud.zip" download_plugins(url=url, target=self.working_path, back_cmd_script='build.sh') def render_template(self, cluster, mixed_vars, task): return super().render_template(cluster, mixed_vars, task) def create_terraform(self): return super().create_terraform() def plan_terraform(self): return super().plan_terraform() def apply_terraform(self): return super().apply_terraform() |
执行
如果都是标准化的,可以直接使用批量创建的方式,我这里考虑非标,所以是for循环
1 2 3 4 5 6 7 8 9 10 11 12 13 14 |
client = AliCloudClient({ "access_key": access_key, "secret_key": secret_key, "region": "cn-shanghai" }) client.init_terraform('alicloud/ecs', {'id': id}) client.render_template('ecs', {'hosts': params}, {'id': id}) client.create_terraform() ret = client.plan_terraform() ret_code, out, err = client.apply_terraform() if ret_code != 0: logger.error('error:{}'.format(err)) return ret_code, out, err |
terraform.tf.j2
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 |
provider "alicloud" { access_key = "${var.access_key}" secret_key = "${var.secret_key}" region = "${var.region}" } {% for host in hosts %} resource "alicloud_instance" "{{host.instance_host_short_name}}" { image_id = "{{host.image_id}}" vswitch_id = "{{host.alicloud_vswitch.id}}" security_groups = [{{host.security_groups_ids}}] availability_zone = "{{ host.availability_zone }}" instance_type = "{{host.instance_type}}" system_disk_size = {{host.system_disk_size}} system_disk_category = "{{host.system_category}}" instance_name = "{{ host.instance_instance_name }}" host_name = "{{ host.instance_host_name }}" instance_charge_type = "{{ host.instance_charge_type }}" } {% endfor %} |
目标样例
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 |
provider "alicloud" { access_key = "${var.access_key}" secret_key = "${var.secret_key}" region = "${var.region}" } resource "alicloud_instance" "devops-Dev-terraform-test2-01" { image_id = "m-uf6xxxxxxxxxxx9wdayy0" vswitch_id = "vsw-uf67xxxxxxxxxxxx42ki6" security_groups = ["sg-uf6hxxxxxxxxxx2c8w"] availability_zone = "cn-shanghai-g" instance_type = "ecs.t5-lc1m1.small" system_disk_size = 100 system_disk_category = "cloud_efficiency" instance_name = "devops-Dev-terraform-test2-01" host_name = "devops-Dev-terraform-test2-01" instance_charge_type = "PostPaid" } |
如果想赏钱,可以用微信扫描下面的二维码,一来能刺激我写博客的欲望,二来好维护云主机的费用; 另外再次标注博客原地址 itnotebooks.com 感谢!
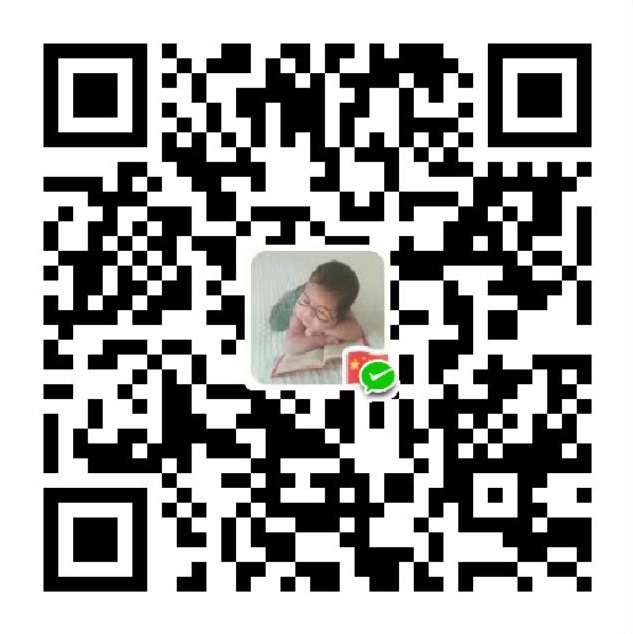