10-13 4 views
1. 安装阿里云域名管理模块
参考:https://develop.aliyun.com/tools/sdk/?spm=a2c4g.11186623.2.9.396c37b4Wlzpx5#/python
1 |
pip install aliyun-python-sdk-domain |
2. 接口使用
https://help.aliyun.com/document_detail/67712.html?spm=a2c4g.11174283.6.674.bcaec8ca90FY8R
3. 代码
以下为个人在项目中的应用,仅供参考
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 |
#!/usr/bin/env python3 # -*- coding: utf-8 -*- # @Author : Eric Winn # @Email : eng.eric.winn@gmail.com # @Time : 2019/10/13 9:32 PM # @Version : 1.0 # @File : domain # @Software : PyCharm import json from django.conf import settings from aliyunsdkcore.client import AcsClient from aliyunsdkdomain.request.v20180129.QueryDomainListRequest import QueryDomainListRequest from aliyunsdkdomain.request.v20180129.QueryDomainByInstanceIdRequest import QueryDomainByInstanceIdRequest from libs.utils import get_logger logger = get_logger(__file__) class Domain: ''' 获取阿里云账户下所有的域名信息,包含域名注册信息、注册商、有效期、联系邮箱、dns Server、状态等信息 参考文档:https://help.aliyun.com/document_detail/67712.html?spm=a2c4g.11174283.6.674.bcaec8ca90FY8R 如果传入access_key_id和access_key_secret则使用传入的access key建立连接 返回此账户下所有的域名信息 ''' def __init__(self, access_key=None, secret=None): self.page_size = 50 self.access_key = access_key if access_key else settings.ALIYUN_ACCESS_KEY_ID self.secret = secret if secret else settings.ALIYUN_ACCESS_KEY_SECRET self.client = AcsClient(self.access_key, self.secret, "cn-hangzhou") self.TotalPageNum = 0 self.TotalItemNum = 0 self.currentPage = [] def __do_action(self, request): try: response = self.client.do_action_with_exception(request) except Exception as e: logger.error(e) return return json.loads(str(response, encoding='utf-8')) def __get_total_page_num(self, PageNum=1): ''' 获取总域名数,及当前页域名列表 :param PageNum: :return: ''' request = QueryDomainListRequest() request.set_accept_format('json') request.set_PageNum(PageNum) request.set_PageSize(int(self.page_size)) response = self.__do_action(request) if response: self.currentPage = response['Data']['Domain'] if PageNum == 1: self.TotalPageNum = int(response['TotalPageNum']) def __get_domainInfo(self, domainIns): ''' 获取域名详细注册信息,同whois查询结果 :return: ''' request = QueryDomainByInstanceIdRequest() request.set_InstanceId(domainIns) return self.__do_action(request) def __translate(self, domain): ''' 字段翻译,只获取需要的部分,便于入库 :param domain: :return: ''' domainInfo = {} domainInfo['name'] = domain['DomainName'] domainInfo['domain_isp'] = 1 domainInfo['domain_id'] = domain['InstanceId'] domainInfo['domain_status'] = int(domain['DomainStatus']) domainInfo['registrant_type'] = int(domain['RegistrantType']) domainInfo['registration_date'] = domain['RegistrationDate'] domainInfo['expiration_date'] = domain['ExpirationDate'] info = self.__get_domainInfo(domain['InstanceId']) domainInfo['nameserver_master'] = info['DnsList']['Dns'][0] domainInfo['nameserver_slave'] = info['DnsList']['Dns'][1] domainInfo['owner'] = info['ZhRegistrantOrganization'] domainInfo['email'] = info['Email'] domainInfo['verification_status'] = info['DomainNameVerificationStatus'] return domainInfo def get_domainListInfo(self): domainListInfo = [] if not self.client: return [] self.__get_total_page_num() for page in range(1, self.TotalPageNum + 1): self.__get_total_page_num(page) domainListInfo.extend(list(map(self.__translate, self.currentPage))) return domainListInfo |
如果想赏钱,可以用微信扫描下面的二维码,一来能刺激我写博客的欲望,二来好维护云主机的费用; 另外再次标注博客原地址 itnotebooks.com 感谢!
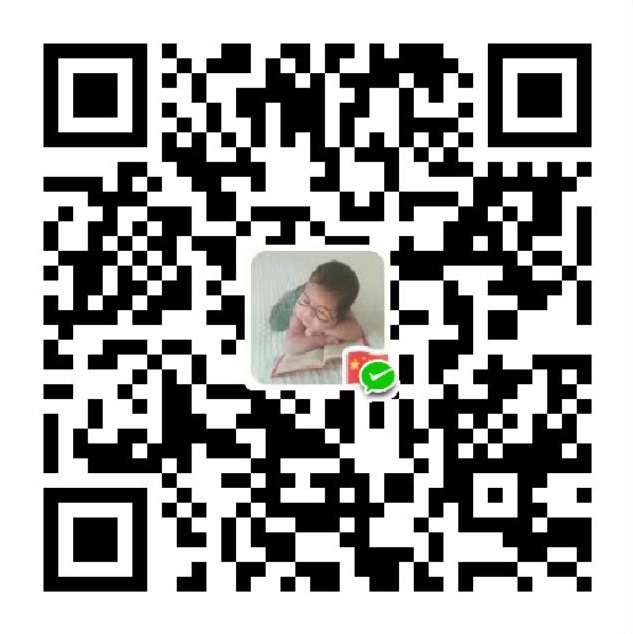