最终效果
第一个文件在上传过程中
目录下第一个文件上传,第二个文件在上传过程中
上传完成及命令执行完成
代码
Python
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 131 132 133 134 135 136 137 138 139 140 141 142 143 144 145 146 147 148 149 150 151 152 153 154 155 156 157 158 159 160 161 162 163 164 165 166 167 168 169 170 171 172 173 174 175 176 177 178 179 180 181 182 183 184 185 186 187 188 189 190 191 192 193 194 195 196 197 198 199 200 201 202 203 204 205 206 207 208 209 210 211 212 213 214 215 216 217 218 219 220 221 222 223 224 225 226 227 228 229 230 231 232 233 234 235 236 237 238 239 240 241 242 243 244 245 246 247 248 249 250 251 252 253 254 255 256 257 258 259 260 261 262 263 264 265 266 267 268 269 270 271 272 273 274 275 276 277 278 279 280 281 282 283 284 285 286 287 |
#!/usr/bin/env python # -*- coding: utf-8 -*- # @Author : Eric Winn # @Email : eng.eric.winn@gmail.com # @Time : 17-5-30 下午1:32 # @Version : 1.0 # @File : sftp # @Software : PyCharm import os import time import logging import paramiko from stat import S_ISDIR from collections import namedtuple from sshtunnel import SSHTunnelForwarder # 定义日志 logging.basicConfig( level='INFO', format='%(asctime)s | %(levelname)s | %(message)s', datefmt='%Y-%m-%d %H:%M:%S', ) class SSH(object): def __init__(self, asset, timeout=30): ''' 请将asset封装成一个namedtuple :param asset: :param timeout: ''' self.ip = asset.ip self.port = asset.port self.username = asset.username self.password = asset.password self.gateway_ip = asset.gateway_ip self.gateway_username = asset.gateway_username self.gateway_password = asset.gateway_password self.gateway_port = asset.gateway_port self.timeout = timeout self.chan = '' self.sftp = '' self.server = '' self.ssh = paramiko.SSHClient() self.Transport = '' # 连接远程主机 def connect(self): try: # 过跳板机,利用跳板机做ssh隧道代理 self.server = SSHTunnelForwarder((self.gateway_ip, self.gateway_port), ssh_password=self.gateway_password, ssh_username=self.gateway_username, remote_bind_address=(self.ip, self.port), local_bind_address=('0.0.0.0', 10022)) self.server.start() except Exception as e: logging.error('({}) {}'.format(self.gateway_ip, e)) return try: print('Connecting to {}'.format(self.ip)) self.Transport = paramiko.Transport(sock=('127.0.0.1', self.server.local_bind_port)) self.Transport.connect(username=self.username, password=self.password) self.sftp = paramiko.SFTPClient.from_transport(self.Transport) except Exception as e: logging.error('({}) {}'.format(self.ip, e)) self.server.close() return try: self.ssh.set_missing_host_key_policy(paramiko.AutoAddPolicy()) self.ssh.connect(hostname='127.0.0.1', port=self.server.local_bind_port, username=self.username, password=self.password, timeout=6) self.chan = self.ssh.invoke_shell() except Exception as e: logging.error('({}) {}'.format(self.ip, e)) self.close() return self.chan # 断开连接 def close(self): print('') print('Disconnecting to {}'.format(self.ip)) try: self.server.stop() self.Transport.close() self.ssh.close() self.chan.close() except: pass # 重启服务 def service_restart(self, service_name): print('开始重启{}'.format(service_name)) self.command('systemctl restart {}'.format(service_name)) # 递归创建目录 def mkdir(self, remote_dir): self.command('mkdir -p {}'.format(remote_dir)) time.sleep(0.3) # 发送命令 def command(self, cmd): try: self.chan.send('{}\n'.format(cmd)) self.get_msg() except Exception as e: logging.error(e) self.close() def get_msg(self): ''' 获取执行结果 :return: ''' try: msg = '' # 通过命令提示符判断是否执行结束 while not msg.endswith('# ') and not msg.endswith('$ '): resp = str(self.chan.recv(1024), 'utf-8') msg += resp print(resp, end='') except Exception as e: logging.error(e) self.close() # 获取目录下所有的文件 def __get_all_files_from_remote_dir(self, remote_dir): all_files = list() if remote_dir[-1] == '/': remote_dir = remote_dir[0:-1] # 获取当前指定目录下的所有目录及文件,包含属性值 files = self.sftp.listdir_attr(remote_dir) for x in files: # remote_dir目录中每一个文件或目录的完整路径 filename = remote_dir + '/' + x.filename # 如果是目录,则递归处理该目录 if S_ISDIR(x.st_mode): all_files.extend(self.__get_all_files_from_remote_dir(filename)) else: all_files.append(filename) return all_files # 获取本地指定目录下的所有文件 def __get_all_files_from_local_dir(self, local_dir): # 保存所有文件的列表 all_files = list() # 获取当前指定目录下的所有目录及文件,包含属性值 files = os.listdir(local_dir) for x in files: # local_dir目录中每一个文件或目录的完整路径 filename = os.path.join(local_dir, x) # 如果是目录,则递归处理该目录 if os.path.isdir(filename): all_files.extend(self.__get_all_files_from_local_dir(filename)) else: all_files.append(filename) return all_files # get单个文件 def get_file(self, remote_file, local_file): logging.debug('') # 获取remote_file的属性值 r_file = self.sftp.lstat(remote_file) if S_ISDIR(r_file.st_mode): logging.error('{} is a directory'.format(remote_file)) return if os.path.isdir(local_file): filename = remote_file.split('/')[-1] local_file = os.path.join(local_file, filename) print('file {} is downloading...'.format(remote_file)) try: self.sftp.get(remote_file, local_file) except Exception as e: logging.error(e) self.close() # put单个文件 def put_file(self, local_file, remote_file): r_file = self.sftp.lstat(remote_file) # 判断是文件还是目录 if S_ISDIR(r_file.st_mode): filename = local_file.split('/')[-1] remote_file = os.path.join(remote_file, filename) if os.path.isdir(local_file): logging.error('{} is a directory'.format(local_file)) return print('file {} is uploading...'.format(local_file)) try: self.sftp.put(local_file, remote_file, callback=self.callback) except Exception as e: logging.error(e) self.close() # get 目录 def get_dir(self, remote_dir, local_dir): all_files = self.__get_all_files_from_remote_dir(remote_dir) for x in all_files: filename = x.split('/')[-1] r_d = os.path.dirname(x).split('/') base_d = remote_dir.split('/') l_d = os.path.join(local_dir, '/'.join(list(set(r_d) ^ set(base_d)))) if not os.path.exists(l_d): os.mkdir(l_d) local_filename = os.path.join(l_d, filename) print('file {} is downloading...'.format(filename)) try: self.sftp.get(x, local_filename) except Exception as e: logging.error(e) self.close() # put目录 def put_dir(self, local_dir, remote_dir): # 去掉路径字符穿最后的字符'/',如果有的话 if remote_dir[-1] == '/': remote_dir = remote_dir[0:-1] # 获取本地指定目录及其子目录下的所有文件 all_files = self.__get_all_files_from_local_dir(local_dir) # 依次put每一个文件 for x in all_files: filename = os.path.split(x)[-1] if os.path.isdir(x): l_d = x.split('/') else: l_d = os.path.dirname(x).split('/') base_d = local_dir.split('/') r_dir = os.path.join(remote_dir, '/'.join(list(set(l_d) ^ set(base_d)))) try: self.sftp.lstat(r_dir) except Exception as e: self.mkdir(r_dir) if os.path.isdir(x): continue remote_filename = os.path.join(r_dir, filename) print('Collecting {}'.format(x)) try: print(' Uploading {} ({:.2f}M)'.format(x, os.path.getsize(x) / 1024 / 1024)) self.sftp.put(x, remote_filename, callback=self.__callback) except Exception as e: logging.error(e) self.close() def __callback(self, send=10, total=10): ''' 需求: 制作传输进度条 1. CallBack方法获取总字节数和已传输字节数 2. 通过计算获取已传输字节数占总字节数的百分比 3. 制作进度条 :param send: :param total: :return: ''' end = '' if send != total else '\n' # 上传进度条 print('\r |{:<50}| {:.2f} M [{:.2f}%]'.format('#' * int(send * 100 / total / 2), send / 1024 / 1024, send * 100 / total), end=end, flush=True) if __name__ == '__main__': asset = namedtuple('asset', [ 'ip', 'username', 'password', 'port', 'gateway_ip', 'gateway_username', 'gateway_password', 'gateway_port' ]) asset.ip = '192.168.1.15' asset.username = 'ServerUser' asset.password = 'RemoteServerPassword' asset.port = 22 # 跳板机配置 asset.gateway_ip = '192.168.1.254' asset.gateway_username = 'GatewayServerUser' asset.gateway_password = 'GatewayServerPassword' asset.gateway_port = 22 ftp = SSH(asset) if ftp.connect(): ftp.put_dir('/tmp/soft', '/tmp/soft') ssh.command('ls -sh /tmp/soft') ftp.close() |
如果想赏钱,可以用微信扫描下面的二维码,一来能刺激我写博客的欲望,二来好维护云主机的费用; 另外再次标注博客原地址 itnotebooks.com 感谢!
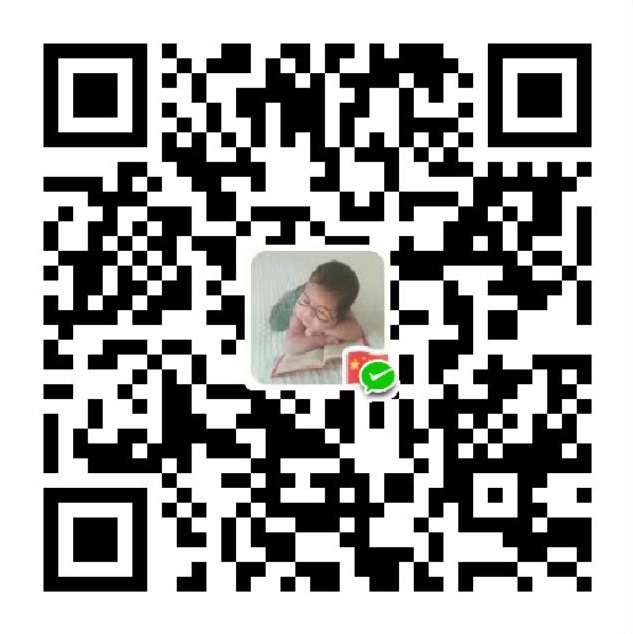